Node.js
Node.js apps can log to Seq with the Bunyan logging framework
The Bunyan library is a logger for JavaScript with wide platform support and good facilities for structured log messages. The bunyan-seq
package is a plug-in for Bunyan that batches log events and posts the to the Seq HTTP ingestion API.
More Seq options for Node.js
See also
pino-seq
for the pino logging library, andwinston-seq
for Winston.
Getting started
First, install both the bunyan
and bunyan-seq
packages.
npm install --save bunyan
npm install --save bunyan-seq
When configuring the Bunyan logger, pass a Seq stream using createStream()
:
let bunyan = require('bunyan');
let seq = require('bunyan-seq');
var log = bunyan.createLogger({
name: 'myapp',
streams: [
{
stream: process.stdout,
level: 'warn',
},
seq.createStream({
serverUrl: 'http://localhost:5341',
level: 'info'
})
]
});
log.info('Hi!');
log.warn({lang: 'fr'}, 'Au revoir');
Configuration
The createStream()
method accepts a configuration object with the following parameters. No parameters are required.
Parameter | Default | Description |
---|---|---|
| The API Key to use when connecting to Seq | |
| 1048576 (1 MiB) | The maximum batch size to send to Seq (should not exceed Settings > System > Raw ingestion payload limit) |
| 262144 (256 kiB) | The maximum event size to send to Seq; larger events will be dumped to stdout (should match Settings > System > Raw event body limit) |
| The Bunyan logging level for the stream | |
| 2000 | The time in milliseconds that the logger will wait for additional events before sending a batch to Seq |
| The Bunyan stream name, which can be used when configuring Bunyan filters | |
| Log to console | A function to receive any errors raised when sending events to Seq |
| false | If |
| http://localhost:5341 | The HTTP endpoint address of the Seq server |
Message templates
You can specify property names as tokens in the log message to control how the event is rendered in Seq:
log.info({user: 'Alice'}, 'Hi, {user}!');
It is not necessary to specify tokens for all properties, but using this technique instead of string formatting will produce more easily machine-readable log events by associating the same template, and thus the same event id, with related events.
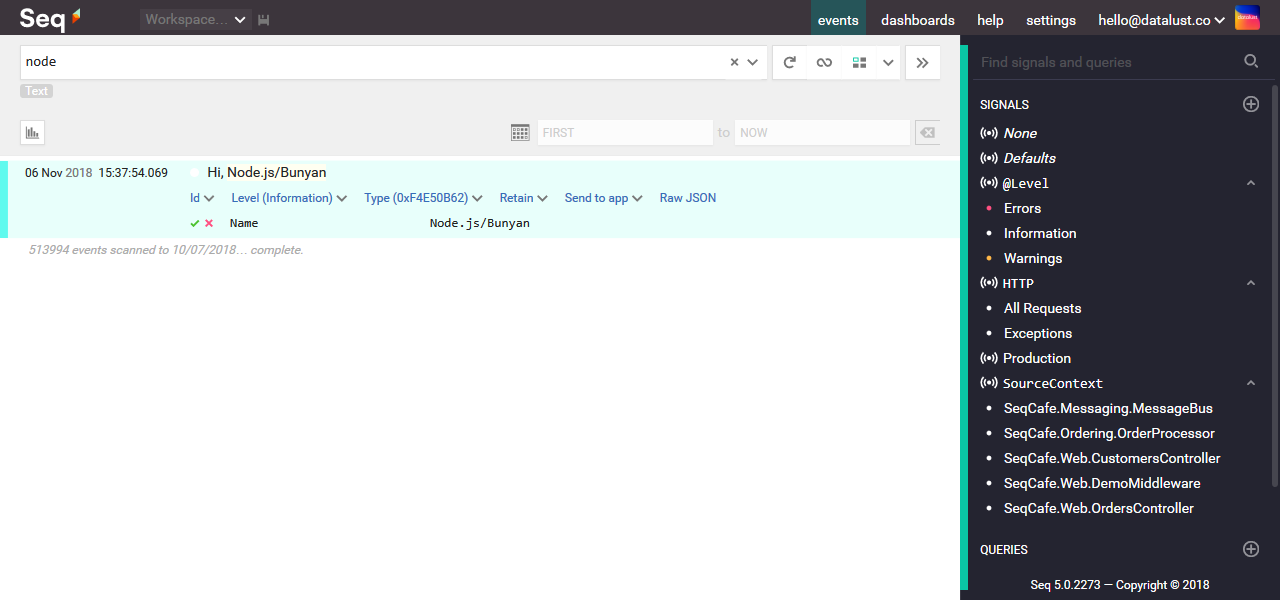
Updated 5 months ago